Quick start
This guide will teach you how to add Inngest to a FastAPI app and run an Inngest function.
💡 Inngest currently supports Django, FastAPI, Flask, and Tornado. If you'd like support for your favorite framework please open an issue on GitHub!
Create an app
⚠️ Use Python 3.10 or higher.
Create and source virtual environment:
python -m venv .venv && source .venv/bin/activate
Install dependencies:
pip install fastapi inngest uvicorn
Create a FastAPI app file:
main.py
from fastapi import FastAPI
app = FastAPI()
Add Inngest
Let's add Inngest to the app! We'll do a few things
- Create an Inngest client, which is used to send events to an Inngest server.
- Create an Inngest function, which receives events.
- Serve the Inngest endpoint on the FastAPI app.
main.py
from fastapi import FastAPI
import inngest
import inngest.fast_api
# Create an Inngest client
inngest_client = inngest.Inngest(
app_id="fast_api_example",
logger=logging.getLogger("uvicorn"),
)
# Create an Inngest function
@inngest_client.create_function(
fn_id="my_function",
# Event that triggers this function
trigger=inngest.TriggerEvent(event="app/my_function"),
)
async def my_function(ctx: inngest.Context, step: inngest.Step) -> str:
ctx.logger.info(ctx.event)
return "done"
app = FastAPI()
# Serve the Inngest endpoint
inngest.fast_api.serve(app, inngest_client, [my_function])
Start your app:
uvicorn main:app --reload
Run Inngest Dev Server
Inngest functions are run using an Inngest server. For this guide we'll use the Dev Server, which is a single-binary version of our Cloud offering. The Dev Server is great for local development and testing, while Cloud is for deployed apps (e.g. production).
Start the Dev Server:
npx inngest-cli@latest dev
After a few seconds, your app and function should now appear in the Dev Server UI:
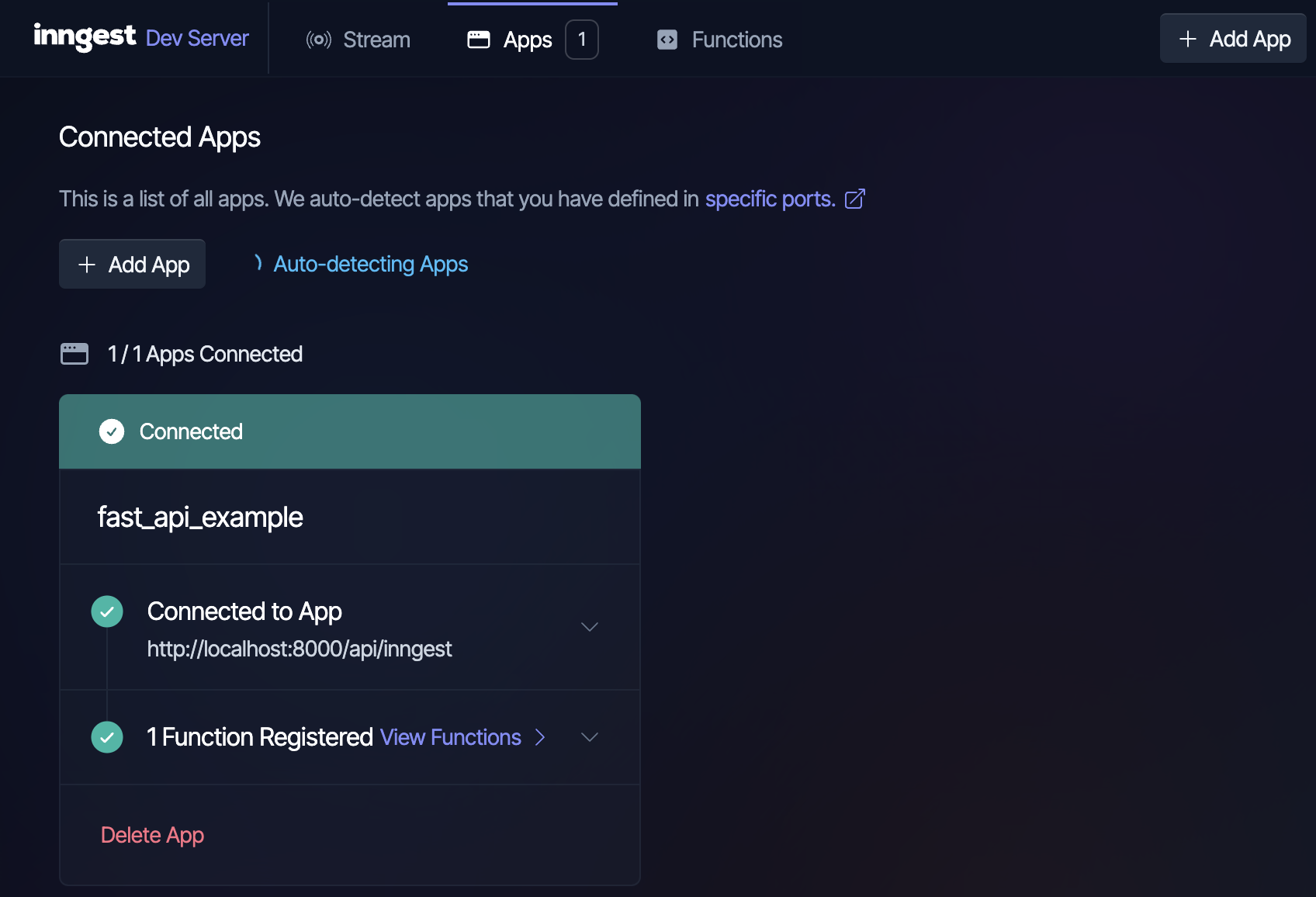
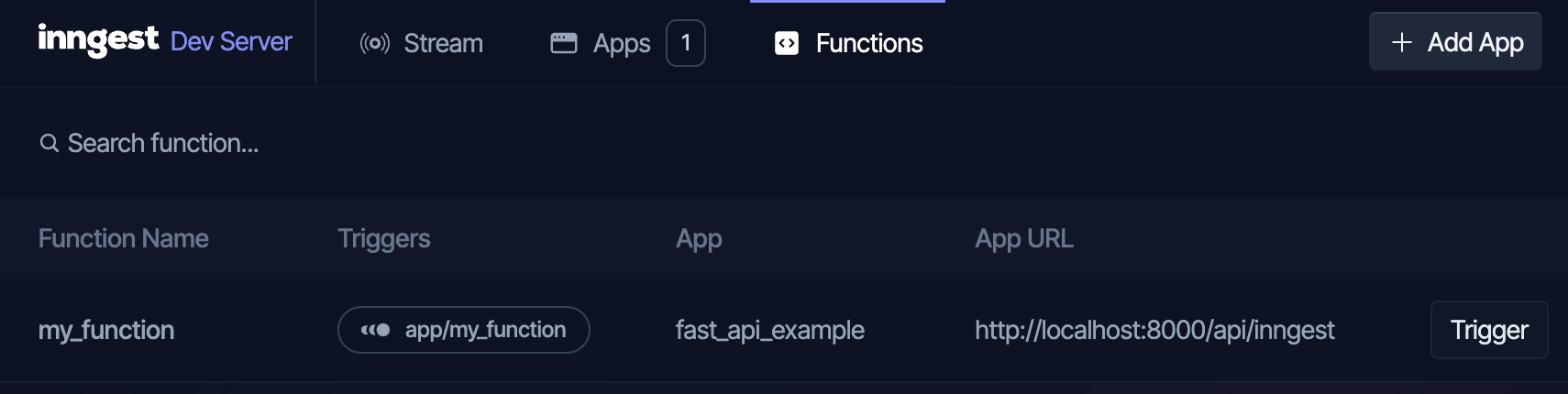
💡 You can sync multiple apps and multiple functions within each app.
Run your function
Click the function's "Trigger" button and a run should appear in the Dev Server stream tab:
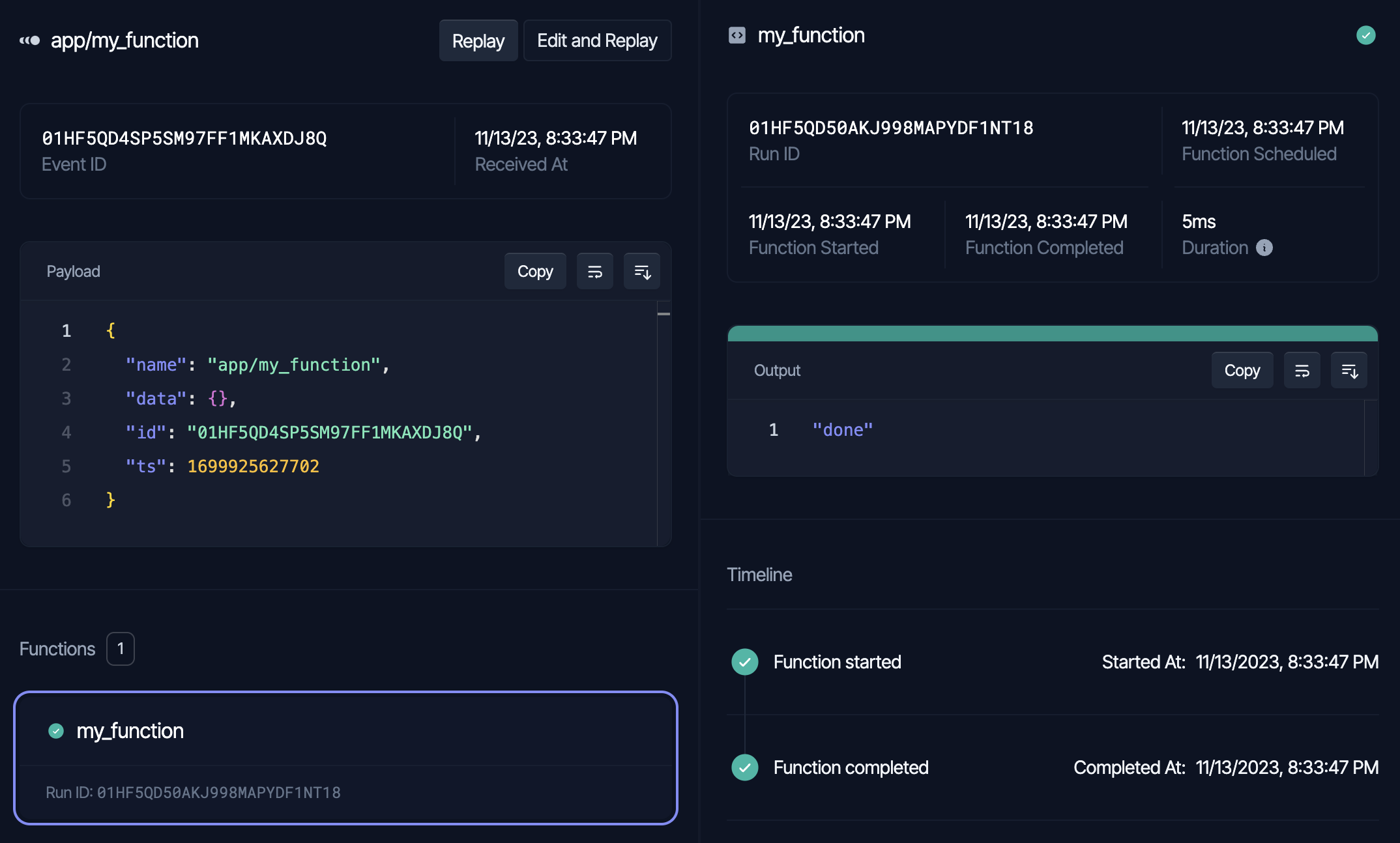